Table of Contents
- When Should Use Redux ?
- Redux main topics
- Action
- Below practical example to understand React Redux easily
- 1) Redux Installation
- 2) src/App.js
- 3) Create folder 'actions' inside src then index.js in it
- 4) Create folder 'reducers' inside src then index.js and upDown.js in it
- 5) Combine reducer inside src/reducers/index.js
- 6) Create store.js inside src folder
- 7) Inside store.js
- 8) inside index.js
- 9) inside App.js
Redux is a free and open-source JavaScript library for managing application state.
The user interface in React is built with Redux. React Redux is a design pattern and framework for maintaining and updating application state through events known as "actions." It acts as a centralised repository for state that must be utilised across your application, with rules guaranteeing that the state may only be altered in predictable ways.
We have also created tutorial on Using React with PHP Laravel framework Create React CRUD with PHP Laravel framework
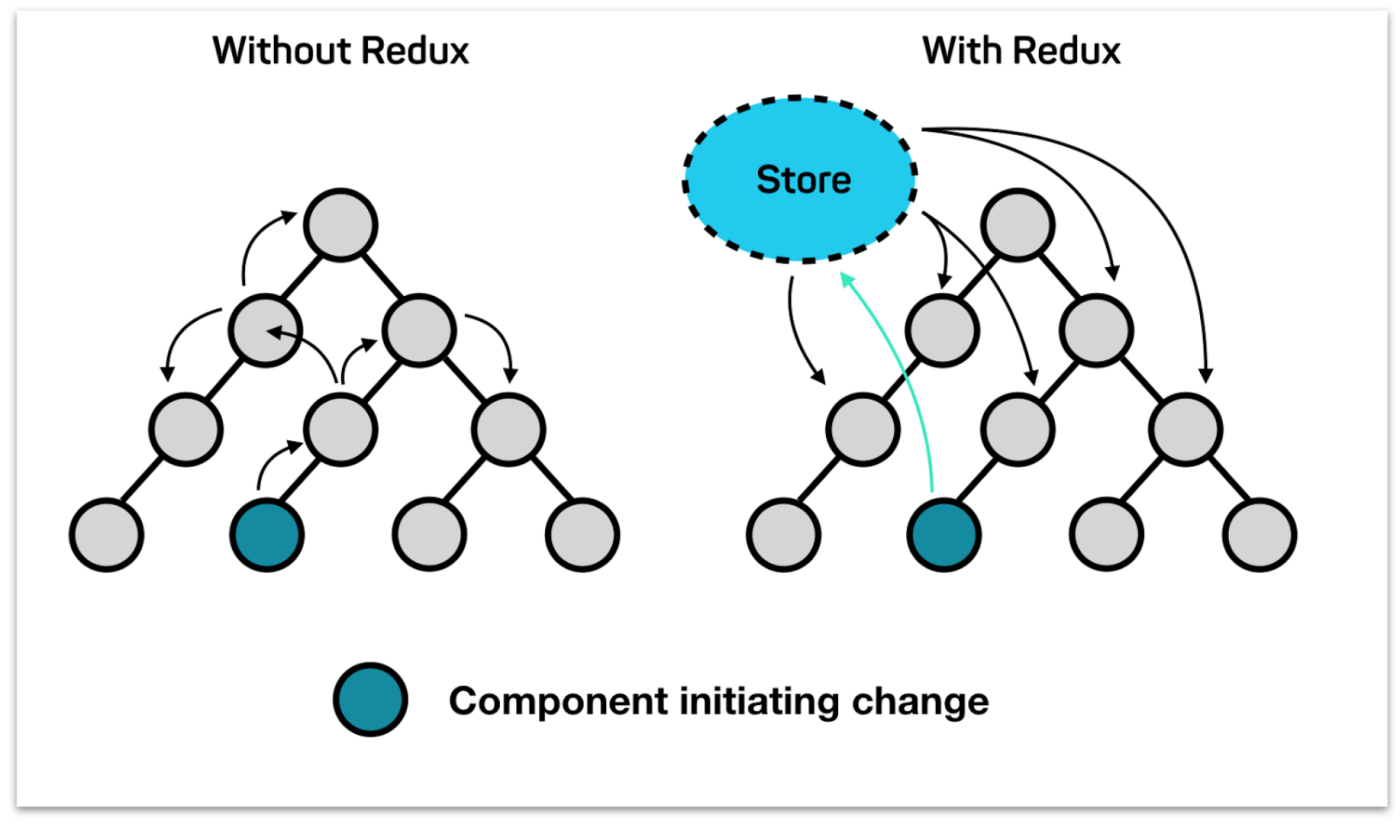
Why Should Use Redux?
Redux assists you with managing "global" state, which is required across several portions of your application. Redux's patterns and tools make it easy to understand when, when, why, and how your application's state is being changed, as well as how your application logic will respond when those changes occur. Redux takes you through the process of building code that is predictable and testable, giving you confidence that your application will function as intended.When Should Use Redux ?
Redux aids with shared state management, but it, like any technology, has compromises. There are more ideas to understand and code to write. Redux is more beneficial in the following situations: - You have a significant quantity of application state that is required in many locations across the app - The app state is constantly updated over time - The logic to update that state may be sophisticated - The app has a medium or large codebase and may be worked on by a large number of people Not all apps require Redux. Take some time to consider the type of app you're creating and which tools will be most useful in solving the difficulties you're working on.Redux main topics
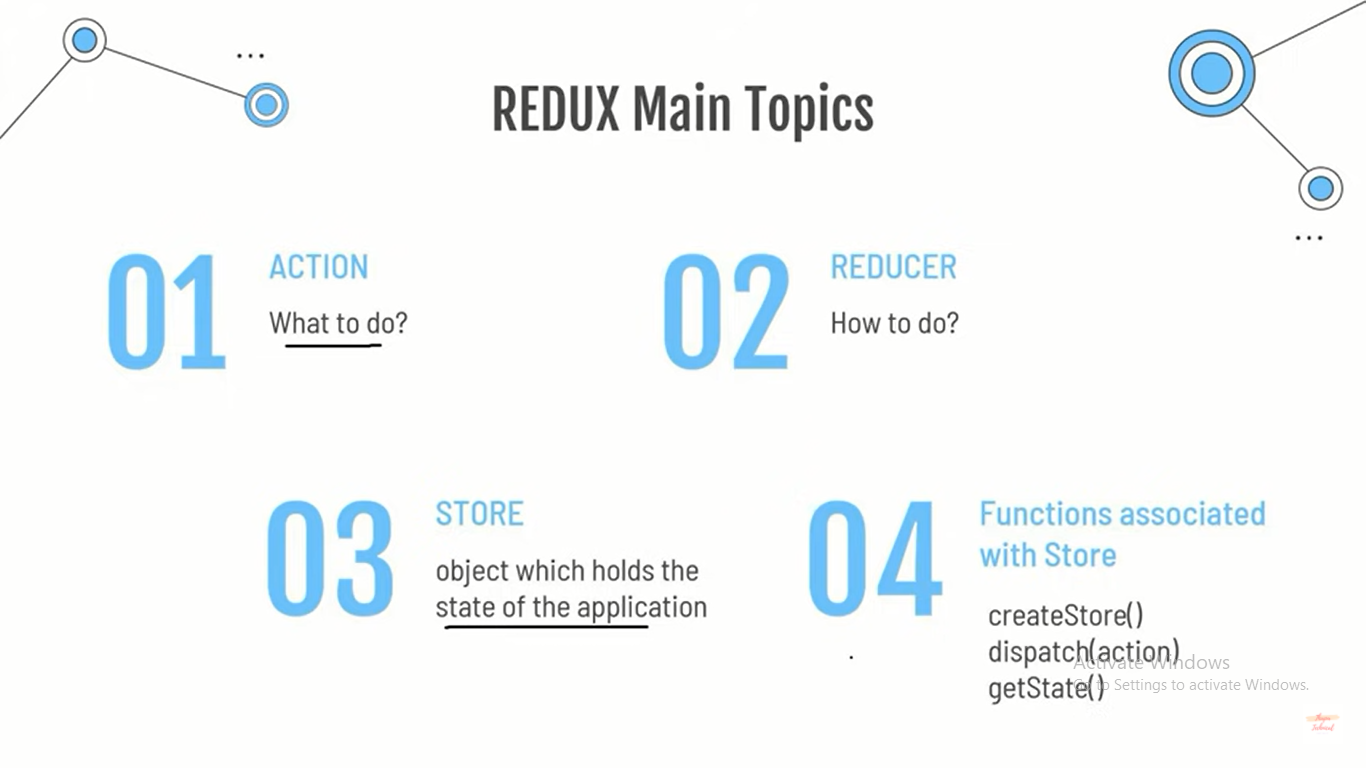
Action
An action is a standard JavaScript object with a type field. Consider an action to be an event that describes something that occurred in the application. The type field should be a string that describes this activity, for as "todos/todoAdded." We often write that type string as "domain/eventName," where the first part is the feature or category to which this action belongs and the second part is the exact item that occurred. Other fields in an action object might provide more information about what happened. We place the information in a field called payload by convention. An example of an action object may be as follows:
Reducer
Simply means 'How to do' A reducer is a function that takes as inputs the current state and an action, chooses how to update the state if required, and returns the new state: (state, action) => newState.
Store
Object that stores the application's state. The Redux store combines the state, actions, and reducers that comprise your app. Note: - In the redux application, there is only one store. - The only way to alter the state is to dispatch an action.Dispatch
The dispatch method is available in the Redux store. The only way to update the state is to use the store method. dispatch() and give an action object as an argument. The store will execute its reducer function and save the new state value inside, and we can access the updated value by calling getState()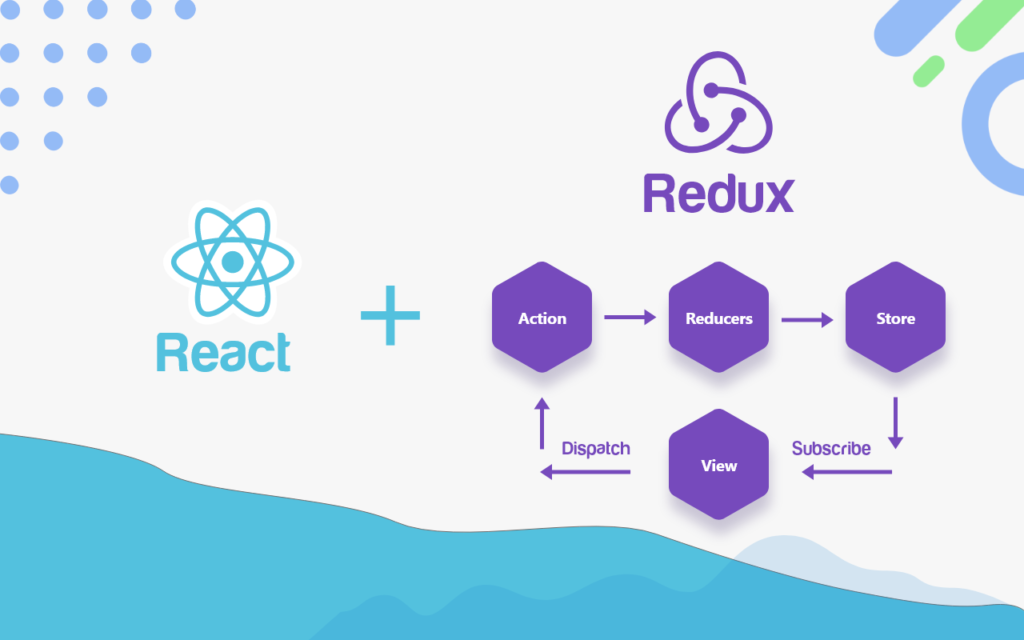
Below practical example to understand React Redux easily
1) Redux Installation
Redux is available as a package on NPM for use with a module bundler or in a Node application:
then
also recommended to install below Redux dev tool in chrome
https://chrome.google.com/webstore/detail/redux-devtools/lmhkpmbekcpmknklioeibfkpmmfibljd?hl=en
We will test later on below topics2) src/App.js
it only contains display of our example that perform number increment and decrement on button click
3) Create folder 'actions' inside src then index.js in it
src/actions/index.js and put below
4) Create folder 'reducers' inside src then index.js and upDown.js in it
src/reducers/index.js src/reducers/upDown.js put below inside upDown.js
5) Combine reducer inside src/reducers/index.js
Put below
6) Create store.js inside src folder
src/store.js and put below
7) Inside store.js
test on Redux dev tool of chrome https://github.com/zalmoxisus/redux-devtools-extension#11-basic-store
8) inside index.js
In this file we imported store, Provider and wrapped App components with Provider. So, our App component and any child component can use the store state.
9) inside App.js
Note: we will use functional component to use Redux hook
Note:
- above 'dispatch' trigger the action
In this way i tried to explain Redux usage in React. You can write your comment if you have any query, I will try to revert soon.
You can also visit here for ReactJs training .